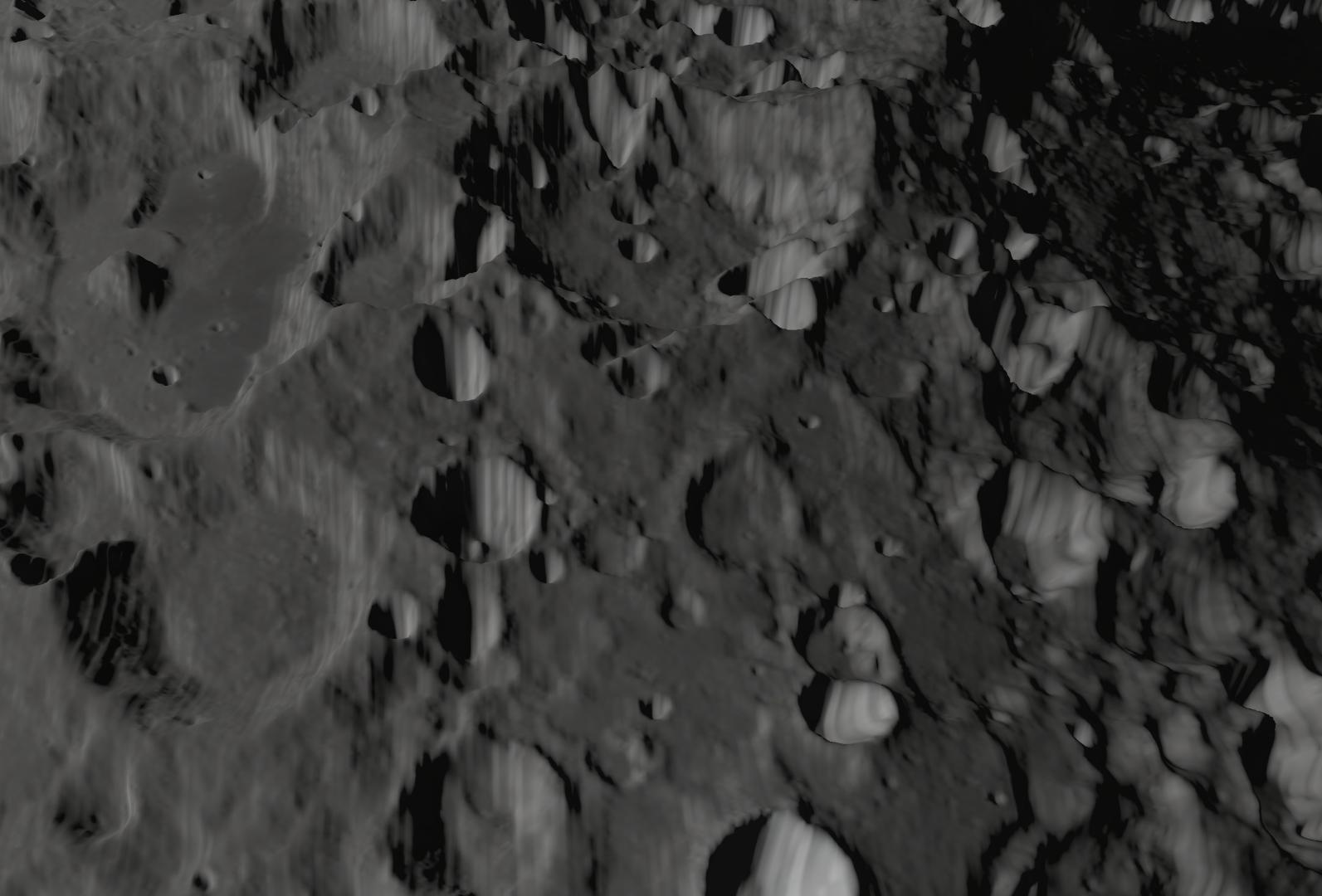
I'm currently implementing Moon terrain with DX12. I use QuadSphere (CubeSphere) and apply tessellation.
Moon is sphere, so we can calculate texture coordinate from polar coordinate. I used the UV for color map and displacement map.
Each map is divided into half horizontally, so I remap texture coordinate. (one texture 23040x11520 -> two texture 11520x11520, 11520x11520)
int texIndex = round(gTexCoord.x);
float2 sTexCoord = float2(texIndex == 0 ? gTexCoord.x * 2 : (gTexCoord.x - 0.5f) * 2.0f, gTexCoord.y);
Everyting works fine, lighting works fine except for the one problem. Lighting is a bit weird. You can see weird stripes on top image.
I guess the problem is coming from normal calculated from displacement map, but not sure. (below code)
What causes the problem? Please help…
// texMap[0] - right color map, texMap[1] - left color map
// texMap[2] - right displacement map, texMap[3] - left displacement map
// Calculate local normal from height map.
float2 offxy = { off.x / nTex.x, off.y / nTex.y };
float2 offzy = { off.z / nTex.x, off.y / nTex.y };
float2 offyx = { off.y / nTex.x, off.x / nTex.y };
float2 offyz = { off.y / nTex.x, off.z / nTex.y };
float s01 = texMap[texIndex + 2].SampleLevel(samLinear, sTexCoord + offxy, 0).r;
float s21 = texMap[texIndex + 2].SampleLevel(samLinear, sTexCoord + offzy, 0).r;
float s10 = texMap[texIndex + 2].SampleLevel(samLinear, sTexCoord + offyx, 0).r;
float s12 = texMap[texIndex + 2].SampleLevel(samLinear, sTexCoord + offyz, 0).r;
float3 va = normalize(float3(size.xy, s21 - s01));
float3 vb = normalize(float3(size.yx, s12 - s10));
float3 localNormal = float3(cross(va, vb) / 2 + 0.5f);
localNormal = localNormal * 2.0f - 1.0f;