I want to display 2 textures on 2 squares. Here's what it looks like.
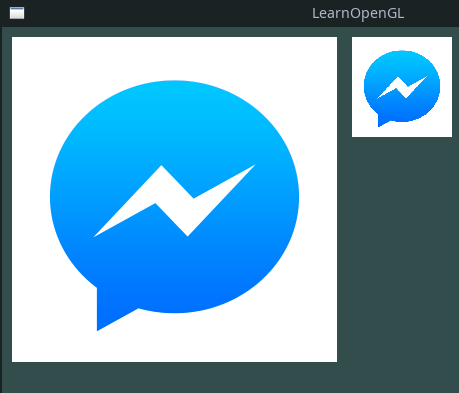
the image size is 325x325. How to make the texture of the right side more sharp.the only way to get good quality is when I use textures and objects with the same size.I'm using PNG images and the following GL methods.
My vertex
#version 430 core
layout(location = 0) in vec2 position;
layout(location = 1) in vec2 texCoord;
layout(location = 2) in float layer;
out vec2 uv;
out float layer_get;
uniform mat4 MVP;
void main()
{
gl_Position = MVP * vec4(position.x, position.y, 0.0, 1.0);
uv = texCoord;
layer_get = layer;
}
My fragment
#version 430 core
out vec4 color;
in vec2 uv;
in float layer_get;
layout (binding=0) uniform sampler2DArray textureArray;
void main()
{
color = texture(textureArray, vec3(uv.x,uv.y, 0));
}
Gl methods
// Create storage for the texture. (1 layer of 325x325 texels)
gl::TexStorage3D(
gl::TEXTURE_2D_ARRAY,
1,
gl::RGBA8, // internal format
325,
325, // WxH
1, // Number of layers
);
let img = image::open(&Path::new("y.png")).unwrap();
let to_rgba = img.to_rgba();
let data = to_rgba.into_vec();
gl::TexSubImage3D(
gl::TEXTURE_2D_ARRAY,
0, // mipmap number
0,
0,
0, //x, y, z
325,
325,
1,
gl::RGBA, // format
gl::UNSIGNED_BYTE, // type
&data[0] as *const u8 as *const c_void, // pointer to data
);
}
I've also heard about mipmap, but I don't understand what it is or how it works... and how should I include it in my texture array
I also heard about Mipmaps. They are smaller, pre-filtered versions of a texture image, But I have absolutely no idea how to include it in my texture array