Hi all.
I created a class named "wall" in my mobile java game. I have a player ship and easly detect collision between player and wall blocks.
My problem is how do i detect collision between wall blocks in same array. I want to check if the blocks intersects with each other. For example one block will carry on other block. Without collision detection i cannot do that.
Collision isnt working at green boxes in same array
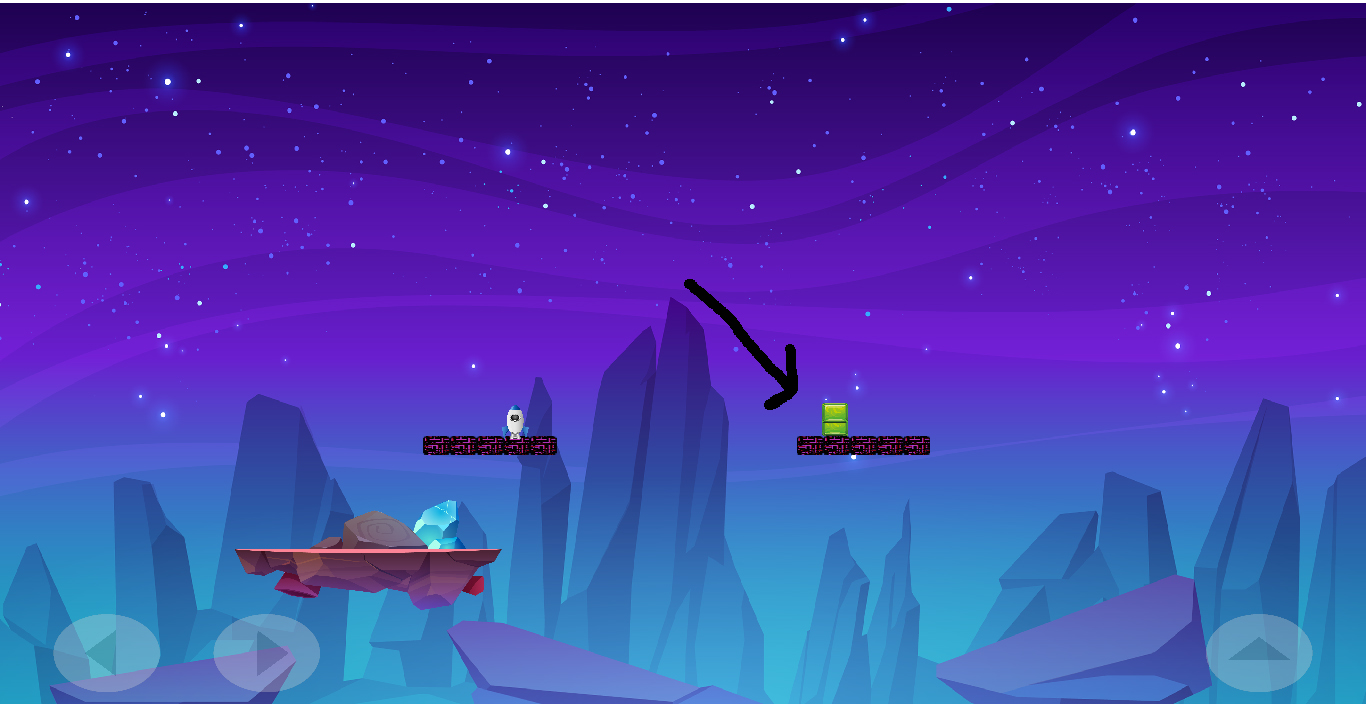
Wall class:
public class Wall {
Vector2 pozisyon = new Vector2();
TextureRegion resim;
Boolean hit;
int wallfall = 1;
public Wall(float x, float y, TextureRegion resim) {
this.pozisyon.x = x;
this.pozisyon.y = y;
this.resim = resim;
}
}
Main code:
/////////////////
private Array <Wall> walls = new Array<Wall>();
////////
for (int i = 0; i < 1; i++) {
for (int q = 0; q < 2; q++) {
walls.add(new Wall(900 + i * 250, 300 + q * 100, wall1));
}
}
//////////
for (Wall wall : walls) {
wallbox.set(wall.pozisyon.x, wall.pozisyon.y, 50, 50);//UPDATE
oyunsayfasi.draw(wall.resim, wall.pozisyon.x, wall.pozisyon.y, 50, 50);//DRAW
}
I tries a code like this
for(int i = walls.size-1; i >= 0; i--) {
walls.get(i);
for(int j = walls.size-1; j >= 0; j--) {
walls.get(j);
if ((walls.get(j).pozisyon.y > walls.get(i).pozisyon.y+50) && (walls.get(j).pozisyon.y+50 < walls.get(i).pozisyon.y)) {
if ((walls.get(j).pozisyon.x > walls.get(i).pozisyon.x+50) && (walls.get(j).pozisyon.x+50 < walls.get(i).pozisyon.x)){
wall.pozisyon.y += 1;
}
}
}
}
But it doesnt work :/ How could i solve this problem? Thanks for every help and idea. Best regards.