Hi,
I am working on implementing animation and I am quite confused on a specific area. I have a turn animation which turns the player but it also has translation data. Once the animation finishes, it snaps back to the original position it started from instead of remaining where it finished the animation. I am wondering how you handle animation translation and how you can keep the animations position as the new player position once the animation has finished.
Currently I am applying the translation data per frame to the Root bone.
// This will find the new rotation and translation data for the current frame
// you get back a pair which gives .first as rotation and .second and translation
std::pair<Quaternion, vec3> updatedPose = NewPose(frame, time_in_seconds, scale, joint->id);
Mat4 rotationMatrixFromQuat = updatedPose.first.ToRotationMatrix();
vec4 offsetFromParent = vec4(jointOffsetX, jointOffsetY, jointOffsetZ, 1.0f);
if(joint->jointName == "Root")
{
offsetFromParent.x = updatedPose.second.x;
/* There is y-translation, but this makes animations go up and down between transitions. I am not sure how to handle this. */
//offsetFromParent.y = updatedPose.second.y;
offsetFromParent.z = updatedPose.second.z;
}
This is the current result I am getting where the animations turns but snaps back to the place it started instead of remaining where it was.
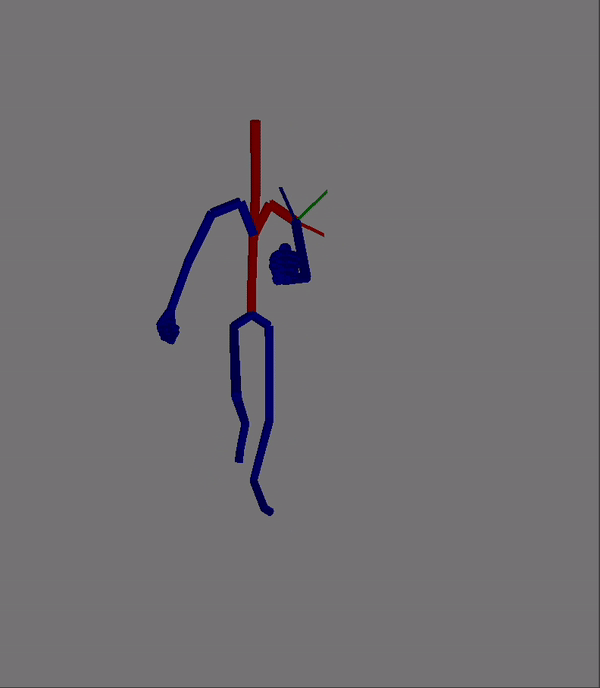