Hi there,
I have had this problem for a while now and it's got me very confused. When I make a swap chain and resize its buffers to the same size as a window's new client area (after a change from a WM_SIZE message) everything is fine.
However I would like to render at full screen size but at a resolution lower than full screen resolution. Just like when you change the resolution settings in a game but it doesn't shrink the window. I've read a lot of the MSDN and couldn't find anything specific to this problem, or if I did, I didn't understand it properly.
AFAIK I can make a swap chain's buffers a certain size, and then have them stretched over larger client area which would give me the effect I want. When I make the swap chain I use the following code:
DXGI_SWAP_CHAIN_DESC sd;
sd.BufferDesc.Width = mClientWidth;
// set to 1280 sd.BufferDesc.Height = mClientHeight;
// set to 720sd.BufferDesc.RefreshRate.Numerator = mRefreshNumerator;
// 1sd.BufferDesc.RefreshRate.Denominator = mRefreshDenominator;
// 60sd.BufferDesc.Format = mBackBufferFormat;
sd.BufferDesc.ScanlineOrdering = DXGI_MODE_SCANLINE_ORDER_UNSPECIFIED;
sd.BufferDesc.Scaling = DXGI_MODE_SCALING_STRETCHED; // was originally DXGI_MODE_SCALING_UNSPECIFIED - 2/3/25 CH
sd.SampleDesc.Count = 1;
sd.SampleDesc.Quality = 0;
sd.BufferUsage = DXGI_USAGE_RENDER_TARGET_OUTPUT;
sd.BufferCount = SwapChainBufferCount;
sd.OutputWindow = mMainWnd;
sd.Windowed = true; // if in doubt use true
sd.SwapEffect = DXGI_SWAP_EFFECT_FLIP_DISCARD;
sd.Flags = DXGI_SWAP_CHAIN_FLAG_ALLOW_MODE_SWITCH;
Note I make use of the DXGI_MODE_SCALING_STRETCHED
flag for the MODE DESC scaling part. I naively hoped this would solve things but it hasn't. I believe you also have to use the DXGI_SWAP_CHAIN_FLAG_ALLOW_MODE_SWITCH
to override some of DXGI's default behaviour to make the effect I'm hoping for possible.
So when a WM_SIZE message occurs I don't resize the swap chain buffers, I just call this:
mSwapChain->SetFullscreenState(true, NULL);
And it mostly works, stretching 1280 x 720 over a 1920 x 1080 display. But it leaves the most terrible flickering near the top of the window:
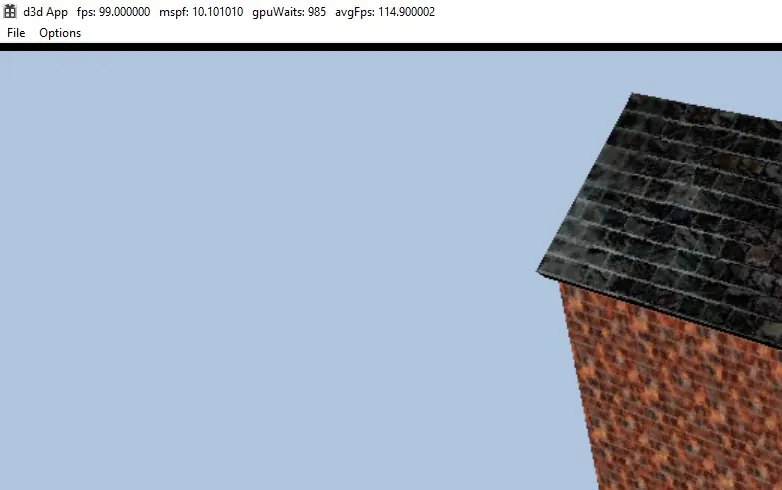
Note the black line at the top just below the menu bar. In practice it flickers a great deal. It looks like two things are competing to paint that portion of the window.
I don't know if I've messed something up in DirectX or if its the Windows side where I'm not instructing Windows to paint the window properly.
I really haven't got a clue and I'd appreciate any advice.
Thanks 🙂