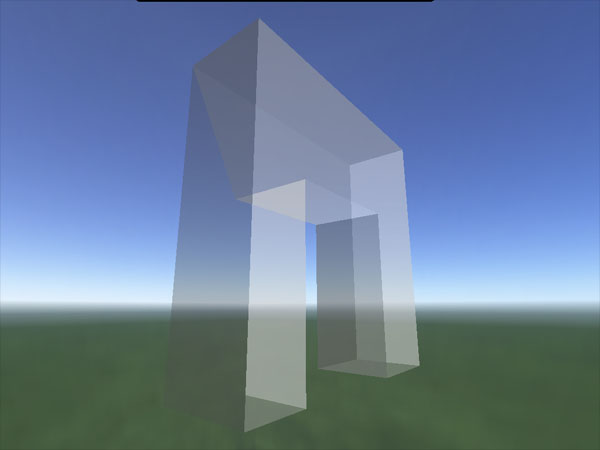
[SOURCE]
struct MCtri
{
int vi[3]; // Vertex index
float dist; // Distance from camera
};
struct MCscene
{
int numVert; // Number of verts
float *vert[3]; // Vertices
int numTri; // Number of triangles
MCtri *tri; // Trianges
float camPos[3]; // Camera position
};
// Compare function
int mcCompare(struct MCtri *elem1, struct MCtri *elem2)
{
if ( elem1->dist < elem2->dist) // If First Structure distance Is Less Than The Second
return -1; // Return -1
else if (elem1->dist > elem2->dist) // If First Structure distance Is Greater Than The Second
return 1; // Return 1
else // Otherwise (If The distance Is Equal)
return 0; // Return 0
}
// Calculate distance between two points in 3d
float mcDistance3d(float[3] ptA, float[3] ptB)
{
float tempa = ptA[MC_X] - ptB[MC_X];
float tempb = ptA[MC_Y] - ptB[MC_Y];
float tempc = ptA[MC_Z] - ptB[MC_Z];
return sqrt((tempa*tempa)+(tempb*tempb)+(tempc*tempc));
}
// Returns the smallest of a group of three numbers (same as min() found in <algorithm>)
float mcSmallest (float A, float B, float C)
{
float smallest;
if (A <= B)
{
if (A <= C) smallest = A;
else smallest = C;
}
else if (B <= C) smallest = B;
else smallest = C;
return smallest;
}
void mcMeasureTriDist( MCscene *s )
{
MCuint32 mc_t;
float dist[3];
for ( mc_t = 0; mc_t < s->numTri; mc_t++ )
{
dist[0] = mcDistance3d( s->camPos, s->tri[mc_t].vi[0]]);
dist[1] = mcDistance3d( s->camPos, s->tri[mc_t].vi[1]]);
dist[2] = mcDistance3d( s->camPos, s->tri[mc_t].vi[2]]);
s->tri[mc_t].dist = mcSmallest( dist[0], dist[1], dist[2] );
}
}
void mcRender( MCscene *s )
{
// Measure distance to camera for each triangle
mcMeasureTriDist(s);
// Sort triangles from back to front
qsort((void *) s->tri, s->numTri, sizeof(struct MCtri), (compfn)mcCompare );
// Render each triangle
for ( mc_t = 0; mc_t < s->numTri; mc_t++)
{
glBegin(GL_TRIANGLES);
glVertex3fv( s->tri[mc_t].vi[0]] );
glVertex3fv( s->tri[mc_t].vi[1]] );
glVertex3fv( s->tri[mc_t].vi[2]] );
glEnd();
}
}
[/SOURCE]