23 hours ago, Wyrframe said:
Sounds like you're trying to writing to a resource to generate one frame, while it's still being used to render another. You're using deferred rendering; can you describe your rendering pipeline, taking particular care to document in what order you perform each step?
Some more imagines to describe the problem:
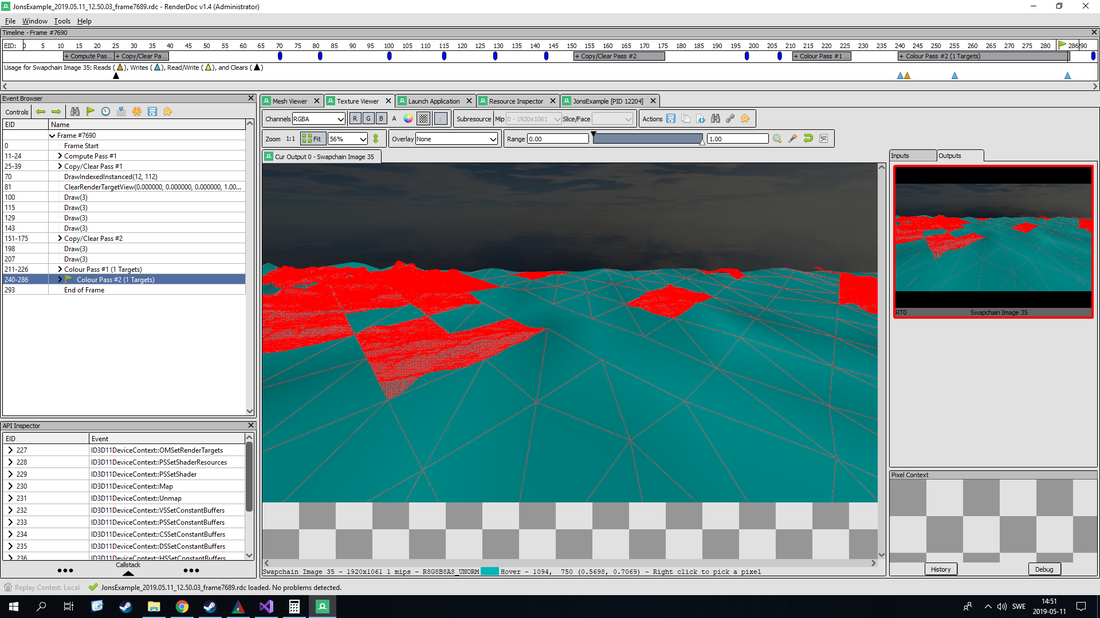
Notice the wierd mix of tessellated and completely non-tessellated patches in the image.
And then at the end frame, everything is non-tessellated:
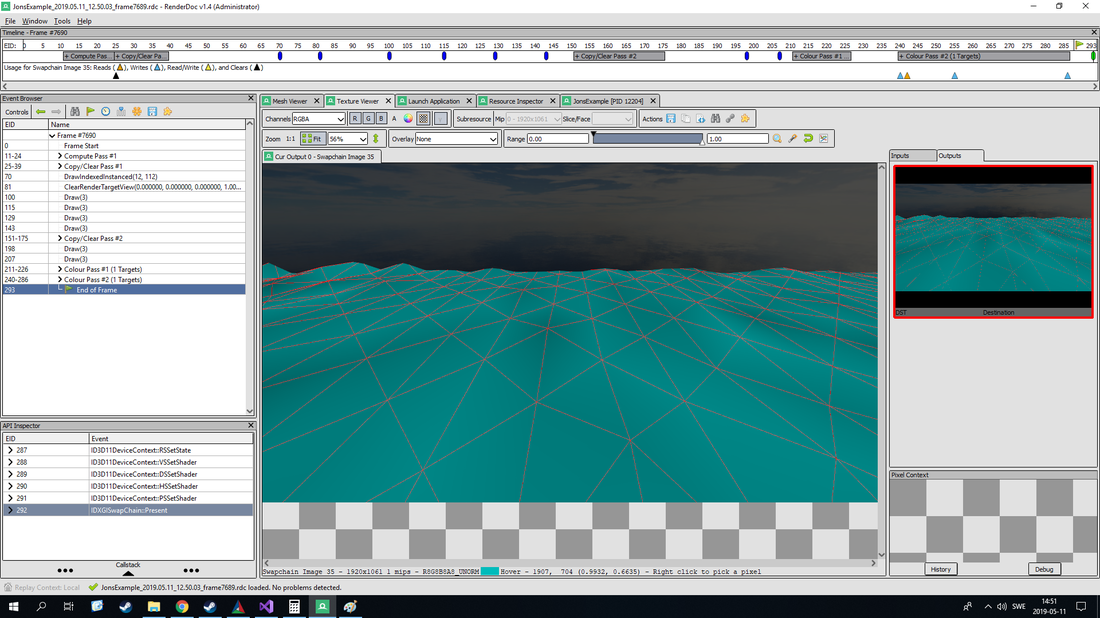
I am not sure how reliable RenderDoc is on the matter though... but the problem remains.
After some more testing, the tearing stops and everything seems to work if I hardcode the tessellation factor in the shader.
This is what it looks like without it, captured sample in renderdoc:
float CalculateTessellationfactor( float3 worldPatchMidpoint )
{
//float cameraToPatchDistance = distance( gWorldEyePos, worldPatchMidpoint );
float scaledDistance = 0.12f;// ( cameraToPatchDistance - gMinZ ) / ( gMaxZ - gMinZ );
scaledDistance = clamp( scaledDistance, 0.0f, 1.0f );
return pow( 2, lerp( 6.0f, 2.0f, scaledDistance ) );
}
And then working sample:
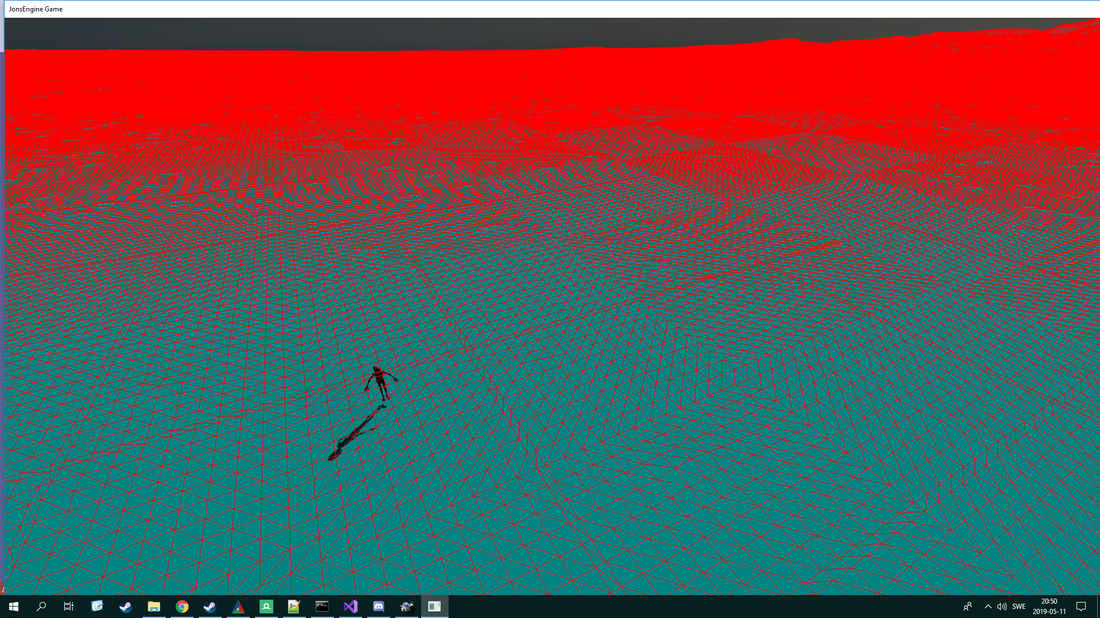
This is just crazy wierd. Here is the complete hull-shader without the edit-fix:
#ifndef TERRAIN_HULL_HLSL
#define TERRAIN_HULL_HLSL
#include "TerrainCommon.hlsl"
#include "Common.hlsl"
float3 ComputePatchMidpoint( float3 corner1, float3 corner2, float3 corner3, float3 corner4 )
{
return ( corner1 + corner2 + corner3 + corner4 ) / 4.0f;
}
float CalculateTessellationfactor( float3 worldPatchMidpoint )
{
float cameraToPatchDistance = distance( gWorldEyePos, worldPatchMidpoint );
float scaledDistance = ( cameraToPatchDistance - gMinZ ) / ( gMaxZ - gMinZ );
scaledDistance = clamp( scaledDistance, 0.0f, 1.0f );
return pow( 2, lerp( 6.0f, 2.0f, scaledDistance ) );
}
PatchTess PatchHS( InputPatch<VertexOut, 12> inputVertices )
{
PatchTess patch;
float3 midPatchMidpoint = ComputePatchMidpoint( inputVertices[ 0 ].mWorldPosition, inputVertices[ 1 ].mWorldPosition, inputVertices[ 2 ].mWorldPosition, inputVertices[ 3 ].mWorldPosition );
float3 edgePatchMidpoint[] = {
ComputePatchMidpoint( inputVertices[ 0 ].mWorldPosition, inputVertices[ 1 ].mWorldPosition, inputVertices[ 4 ].mWorldPosition, inputVertices[ 5 ].mWorldPosition ),
ComputePatchMidpoint( inputVertices[ 1 ].mWorldPosition, inputVertices[ 2 ].mWorldPosition, inputVertices[ 6 ].mWorldPosition, inputVertices[ 7 ].mWorldPosition ),
ComputePatchMidpoint( inputVertices[ 2 ].mWorldPosition, inputVertices[ 3 ].mWorldPosition, inputVertices[ 8 ].mWorldPosition, inputVertices[ 9 ].mWorldPosition ),
ComputePatchMidpoint( inputVertices[ 3 ].mWorldPosition, inputVertices[ 0 ].mWorldPosition, inputVertices[ 10 ].mWorldPosition, inputVertices[ 11 ].mWorldPosition )
};
float midPatchTessFactor = CalculateTessellationfactor( midPatchMidpoint );
float edgePatchTessFactors[] = {
CalculateTessellationfactor( edgePatchMidpoint[ 3 ] ),
CalculateTessellationfactor( edgePatchMidpoint[ 0 ] ),
CalculateTessellationfactor( edgePatchMidpoint[ 1 ] ),
CalculateTessellationfactor( edgePatchMidpoint[ 2 ] )
};
patch.mEdgeTess[ 0 ] = min( midPatchTessFactor, edgePatchTessFactors[ 0 ] );
patch.mEdgeTess[ 1 ] = min( midPatchTessFactor, edgePatchTessFactors[ 1 ] );
patch.mEdgeTess[ 2 ] = min( midPatchTessFactor, edgePatchTessFactors[ 2 ] );
patch.mEdgeTess[ 3 ] = min( midPatchTessFactor, edgePatchTessFactors[ 3 ] );
patch.mInsideTess[ 0 ] = midPatchTessFactor;
patch.mInsideTess[ 1 ] = midPatchTessFactor;
return patch;
}
[domain("quad")]
[partitioning("fractional_odd")]
[outputtopology("triangle_ccw")]
[outputcontrolpoints(4)]
[patchconstantfunc("PatchHS")]
HullOut hs_main( InputPatch<VertexOut, 12> verticeData, uint index : SV_OutputControlPointID )
{
HullOut ret;
ret.mWorldPosition = verticeData[ index ].mWorldPosition;
ret.mTexcoord = verticeData[ index ].mTexcoord;
return ret;
}
#endif
cbuffer PerFrameCB : register(CBUFFER_REGISTER_PER_FRAME)
{
float4x4 gFrameViewProj;
float4x4 gFrameView;
float4x4 gFrameInvView;
float4x4 gFrameInvProj;
float3 gWorldEyePos;
float gMinZ;
float gMaxZ;
};
and inspecting the constant-buffer it looks like this:
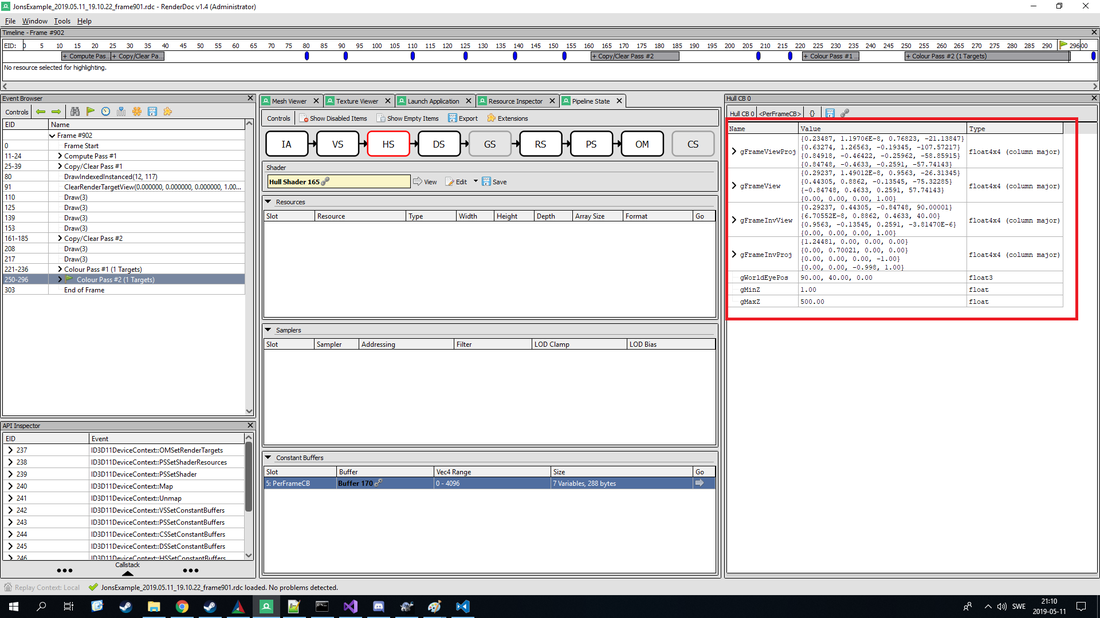
The contents looks legit.
Again, the problem seems localized to this shader, but it might very well be a well-obscured problem.. it's bizare because I use constant buffers all the time and they work just fine. The same constant buffer contains other data like transformation matrices that work just fine.