Hi I am trying to implement ray-casting in my engine so that I can pick objects in 3D. I am able to cast rays by clicking the screen and they are projected into the world with relatively ok accuracy in terms of their origin point. However, the problem is that no matter which direction I cast the ray, it always goes through the origin of the world (0,0,0), see the screenshot below:
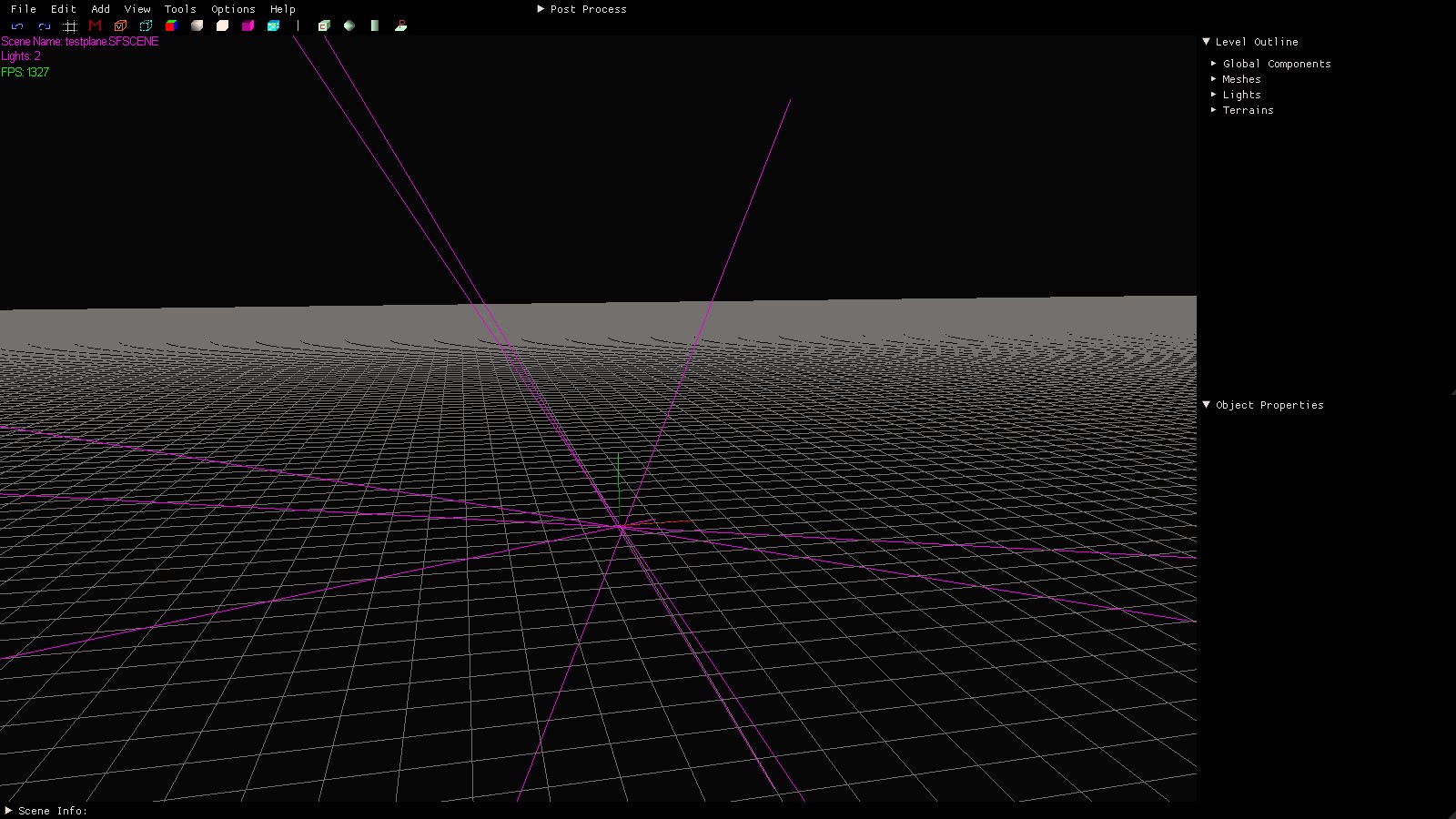
As you can see, all the rays diverge to the middle of the world, even though that is not the point on the screen where I clicked. Below is the code I use to generate the ray. Note that I am not normalizing the direction vector because I found that when I do that, the ray is cut off and doesn't go all the way to the middle. What am I doing wrong? Thanks
D3DXVECTOR3 rayStart, rayDir;
rayStart = m_Camera->GetPosition();
cout << m_screenWidth << " " << m_screenHeight << endl;
rayDir.x = ((2.0f * (float)mouseX) / (float)m_screenWidth) - 1.0f;
rayDir.y = ((2.0f * (float)mouseY) / (float)m_screenHeight) - 1.0f;
rayDir.z = 1.0f;
D3DXMATRIX viewMatrix, projectionMatrix, inverseMatrix;
m_Camera->GetViewMatrix(viewMatrix);
m_D3D->GetProjectionMatrix(projectionMatrix);
D3DXMatrixMultiply(&inverseMatrix, &viewMatrix, &projectionMatrix);
D3DXMatrixInverse(&inverseMatrix, NULL, &inverseMatrix);
D3DXVec3TransformNormal(&rayDir, &rayDir, &inverseMatrix);
//D3DXVec3Normalize(&rayDir, &rayDir);
Line* line = new Line;
line->Initialize(m_D3D->GetDevice(), 0, rayStart.x, rayStart.y, rayStart.z, rayDir.x, rayDir.y, rayDir.z, 1.0f, 0.0f, 1.0f);
lines.push_back(line);
for (int i = 0; i < meshes.size(); i++) {
bool intersect = false;
intersect = TavianAABBIntersect(rayStart, rayDir, meshes[i]);
if (intersect) {
selected = meshes[i];
break;
}
}