I know something about shaders, I have written some very basic shaders. This is the most advanced shader I have written so far.
It's been several days since I conceived the idea of trying to recreate this shader (image below), but I still can't wrap my head around on how to approach it.
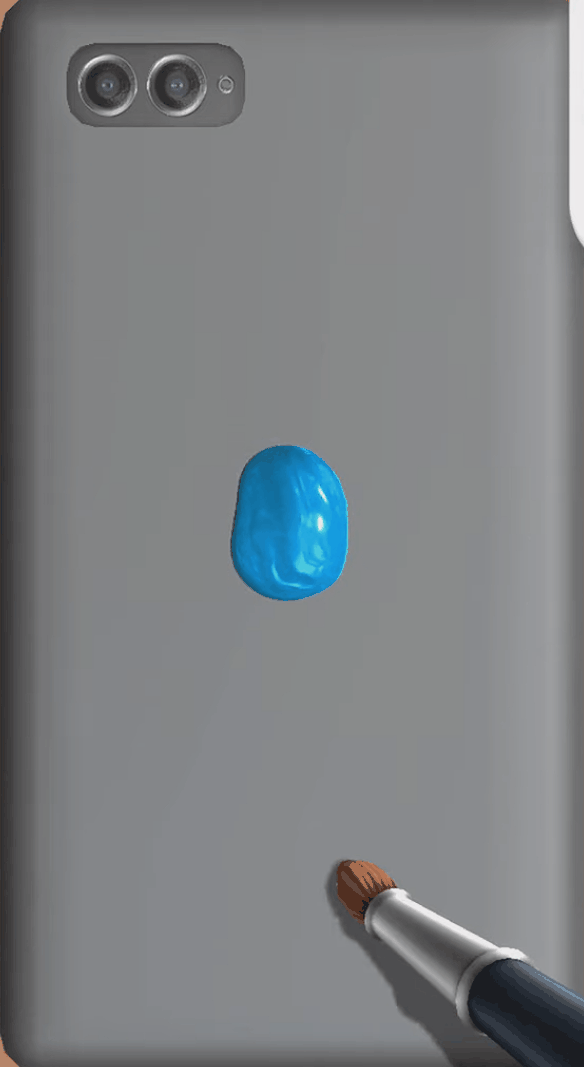
I figured out I can hide part of the object by making the texture fully transparent and then, after player moves the brush across the object, project the brush on to the object and reveal that part of the texture.
Shader "Custom/Masked Transparent" {
Properties {
_Color ("Main Color", Color) = (1,1,1,1)
_MainTex ("Base (RGB) Trans (A)", 2D) = "white" {}
_MaskTex("Mask Texture", 2D) = "white" {}
}
SubShader {
Tags {"Queue"="Transparent" "IgnoreProjector"="True" "RenderType"="Transparent"}
LOD 200
CGPROGRAM
#pragma surface surf Lambert alpha:fade
sampler2D _MainTex;
sampler2D _MaskTex;
fixed4 _Color;
struct Input {
float2 uv_MainTex;
};
void surf (Input IN, inout SurfaceOutput o) {
fixed4 main_color = tex2D(_MainTex, IN.uv_MainTex);
fixed4 mask_color = tex2D(_MaskTex, IN.uv_MainTex);
o.Albedo = main_color.rgb;
o.Alpha = mask_color.r;
}
ENDCG
}
}
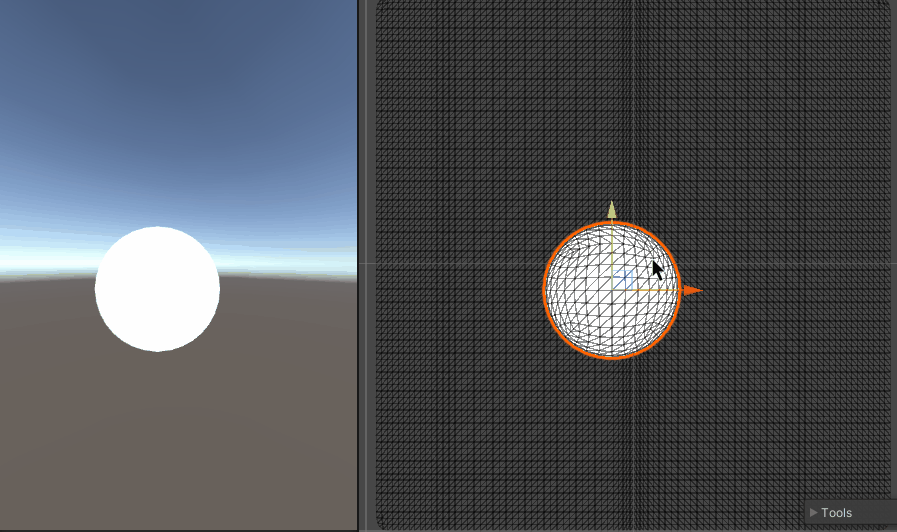
But this implementation lacks the perceived height and liquidity you can observe in the original shader . How do I implement the effect of smearing acrylic paint? Any advice, resources, tutorials and maybe there's ready to use assets - anything is welcome! Thanks!
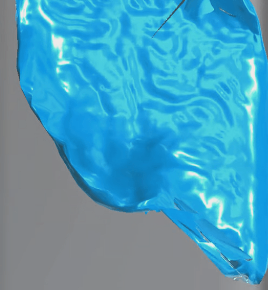
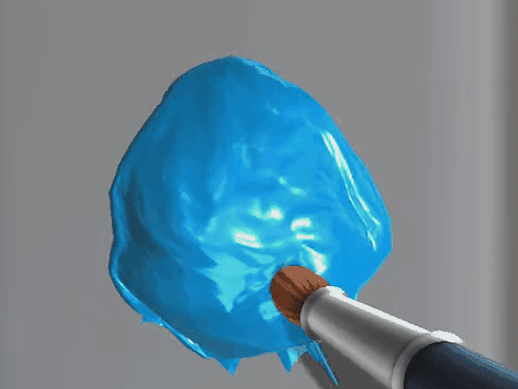