Direct3D and OpenGL are competing application programming interfaces (APIs) which can be used in applications to render 2D and 3D computer graphics. They are the two most popular graphics APIs utilized in game development. In some cases the development team could decide to move from OpenGL to Direct3D, or from Direct3D to OpenGL or even provides a multirendering solution. And to make the task easy we need a good documentation about the API mapping between the two API. Another solution could be to discover the API mapping from an existing source code. Irrlicht 3D engine is a good candidate for this purpose. it implements both DirectX and OpenGL renders.
The Irrlicht Engine is an open-source high-performance realtime 3D engine written in C++. It is completely cross-platform, using D3D, OpenGL and its own software renderers. CppDepend and CQLinq will be used to help us to identify some code elements from the source code. The Irrlicht rendering logic is implemented in the irr::video namespace, and the base interface for material rendering is irr::video::IMaterialRender. Let's search for all the classes implementing directly the irr::video::IMaterialRender interface.
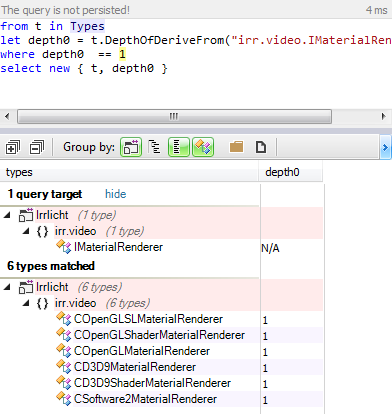
Both DirectX and OpnGL renders are implemented. the COpenGLSLMaterialRenderer, COpenGLShaderMaterialRenderer, CD3D9ShaderMaterialRenderer and CD3D9MaterialRenderer are the base classes for all the other rendering classes. OpenGL renders Let's search for all classes inheriting from COpenGLSLMaterialRenderer and COpenGLShaderMaterialRenderer directly or indirectly:
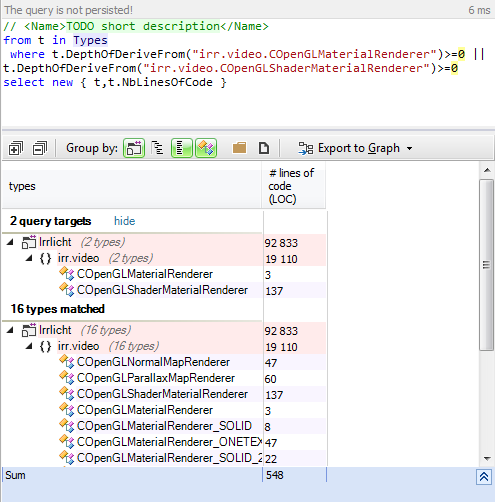
DirectX renders Let's search for all classes inheriting from CD3D9ShaderMaterialRenderer and CD3D9MaterialRenderer directly or indirectly:
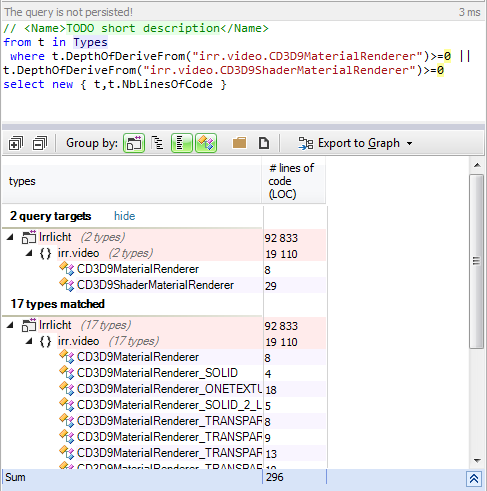
How Irrlicht could help us for the mapping? Let's suppose that you need to migrate a code from OpenGL to Direct3D implementation. And in our code the function glTexEnvi is used and we want to know the equivalent in Direct3D. First Step: Search where in Irrlicht the function is used.
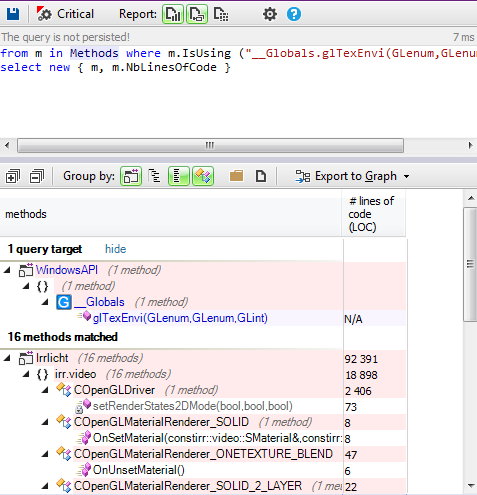
Choose one method using it. Let's take as example OnSetMaterial in COpenGLMaterialRenderer_SOLID. Here' its implementation
virtual void OnSetMaterial(const SMaterial& material, const SMaterial& lastMaterial, bool resetAllRenderstates, IMaterialRendererServices* services) _IRR_OVERRIDE_
{
if (Driver->getFixedPipelineState() == COpenGLDriver::EOFPS_DISABLE)
Driver->setFixedPipelineState(COpenGLDriver::EOFPS_DISABLE_TO_ENABLE);
else
Driver->setFixedPipelineState(COpenGLDriver::EOFPS_ENABLE);
Driver->disableTextures(1);
Driver->setBasicRenderStates(material, lastMaterial, resetAllRenderstates);
if (resetAllRenderstates || (material.MaterialType != lastMaterial.MaterialType))
{
// thanks to Murphy, the following line removed some
// bugs with several OpenGL implementations.
glTexEnvi(GL_TEXTURE_ENV, GL_TEXTURE_ENV_MODE, GL_MODULATE);
}
}
Second step: Find the equivalent in Direct3D Irrlicht implements both OpenGL and Direct3D, and for each rendering class from Direct3D, there's an equivalent for OpenGL. The equivalent of COpenGLMaterialRenderer_SOLID class is CD3D9MaterialRenderer_SOLID. Here's the implementation of OnSetMaterial from the Direct3D render:
virtual void OnSetMaterial(const SMaterial& material, const SMaterial& lastMaterial, bool resetAllRenderstates, IMaterialRendererServices* services) _IRR_OVERRIDE_
{
services->setBasicRenderStates(material, lastMaterial, resetAllRenderstates);
if (material.MaterialType != lastMaterial.MaterialType || resetAllRenderstates)
{
setTextureColorStage(pID3DDevice, 0, D3DTA_TEXTURE, D3DTOP_MODULATE, D3DTA_DIFFUSE);
}
pID3DDevice->SetTextureStageState(1, D3DTSS_COLOROP, D3DTOP_DISABLE);
}
Discover all the OpenGL and Direct3D functions used in Irrlicht
Fortunatly Irrlicht uses many functions from both APIs, and you will certainly find most of your Direct3D or OpenGL functions used in your code. Let's take as example irr::video::CD3D9Driver and search for the Direct3D functions used:
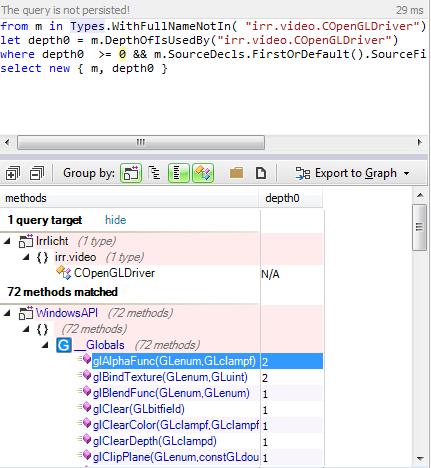
And we can do the same with the irr::video::COpenGLDriver class
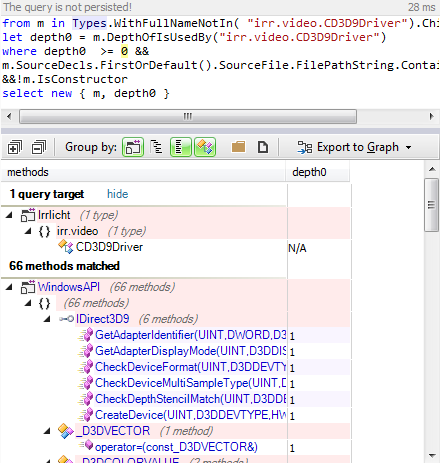
Conclusion
Irrlicht is a good candidate to discover how to map functions between the two APIs. This 3D engine is mature and very well implemented. You can easily search for an API function and find how it's implemented for the other one.
I have used OpenGL for years and I consider myself fairly well versed in some Direc3D versions as well. I don't understand how those evaluations can be relevant. The reported snippets are engine-dependent and don't map to any operation I recognize.
I find this cutting it short.