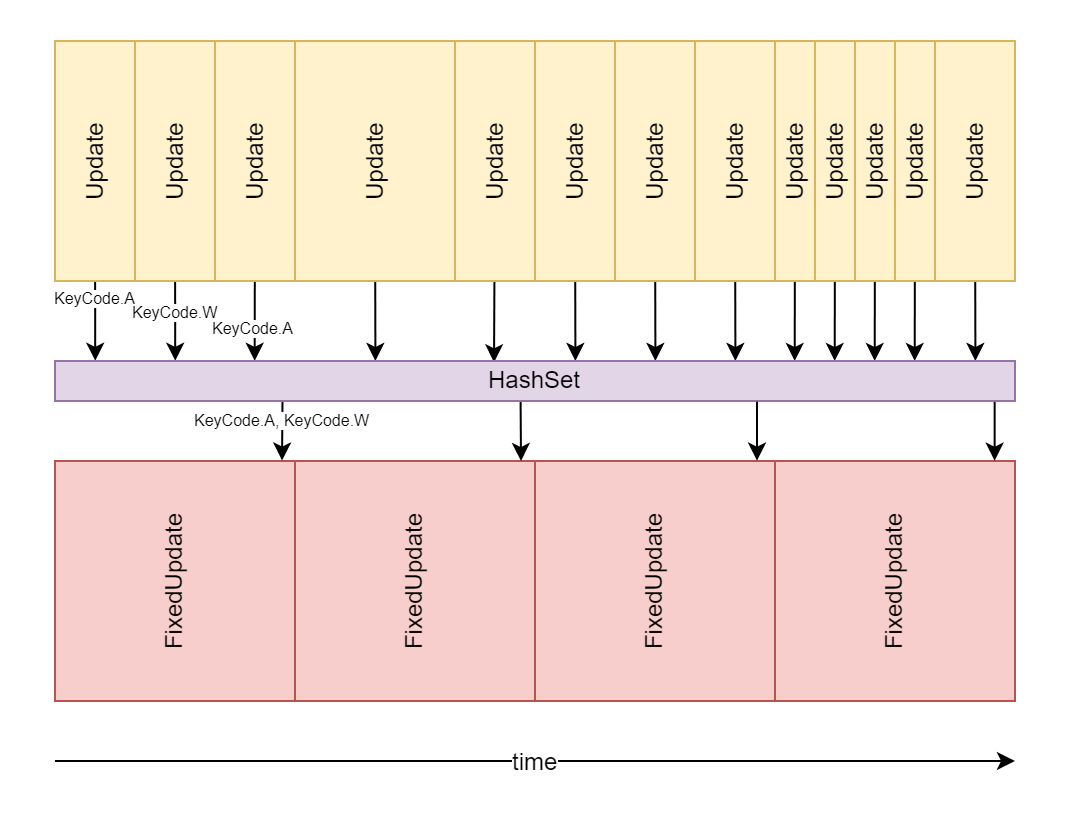
Case, when:
- you want to react to inputs in FixedUpdate method
- but collecting inputs in FixedUpdate method, misses some inputs, like quick tap on a keyboard or quick mouse clicks
- so, you need to collect inputs in Update method and transfer those to FixedUpdate method.
One way to do so is to use HashSet. Collect input states, like whether key X is pressed in Update method and put those in HashSet, later to be used in FixedUpate method. With HashSet you do not have to worry about handling cases when user manages to press, say, key X, multiple times between invocations of FixedUpdate method; HashSet is a Set which, by definition, does not (and will not) contain more than one of same element.
Here is a code example:
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class InputsTransfer : MonoBehaviour
{
private HashSet<KeyCode> keyboardInputs;
// Start is called before the first frame update
void Start()
{
keyboardInputs = new HashSet<KeyCode>();
}
private void CollectKeyboardInputs()
{
if (Input.GetKey(KeyCode.W)) keyboardInputs.Add(KeyCode.W);
if (Input.GetKey(KeyCode.A)) keyboardInputs.Add(KeyCode.A);
if (Input.GetKey(KeyCode.S)) keyboardInputs.Add(KeyCode.S);
if (Input.GetKey(KeyCode.D)) keyboardInputs.Add(KeyCode.D);
}
private void ProcessInputs()
{
if (keyboardInputs.Contains(KeyCode.W)) Debug.Log("Move forward");
if (keyboardInputs.Contains(KeyCode.A)) Debug.Log("Move left");
if (keyboardInputs.Contains(KeyCode.S)) Debug.Log("Move backward");
if (keyboardInputs.Contains(KeyCode.D)) Debug.Log("Move right");
keyboardInputs.Clear();
}
void Update()
{
CollectKeyboardInputs();
}
void FixedUpdate()
{
ProcessInputs();
}
}