Before starting with more complicated staff, lets try what we have with another similar mini-game.
In “Secret” you need to find the code in some number of attempts. To help you, the game shows how many digits are in the right position and how many are in wrong positions.
Example of a play.
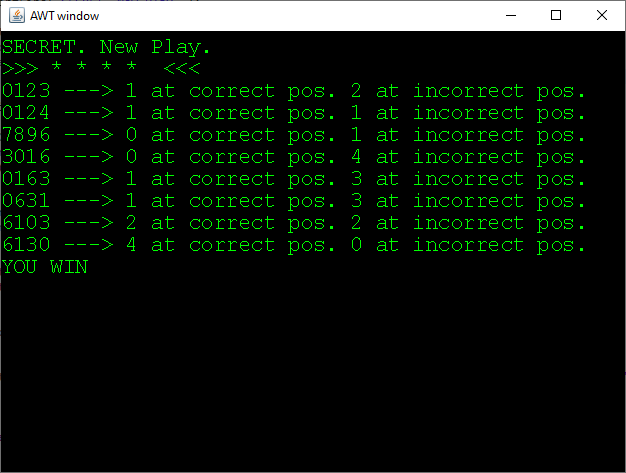
The game loop is very similar to the hungman mini-game.
package manager;
import gateways.IOFactory;
import secret.Play;
public class Secret {
public static void main(String[] args) {
IIOFactory factory = new IOFactory();
//IInput in = factory.makeConsoleInput();
IInput in = factory.makeCanvasAWTInput();
//IOutput out = factory.makeConsoleOutput();
IOutput out = factory.makeCanvasAWTOutput();
out.printLine("SECRET. New Play.");
int attempts = 8;
int secretLength = 4;
Play secret = new Play(secretLength);
String word = secret.getHiddenSecret('*');
out.printLine(">>> " + word + " <<<");
// 2. Game loop
boolean success = false;
while (attempts > 0 && !success) {
// 2.1 User input
String str = in.readString();
str = str.substring(0, secretLength);
// 2.2 Logic check
int correctPosition = secret.checkCorrectPosition(str);
int incorrectPosition = secret.checkIncorrectPosition(str);
// 2.3 Win/Lose check
success = secret.isCorrectSecret(str);
// 2.4 Output
if ( success ) {
out.printLine(str + " ---> " + correctPosition + " at correct pos. " + incorrectPosition + " at incorrect pos.");
out.printLine("YOU WIN");
} else {
attempts--;
if ( attempts <= 0 ) {
out.printLine("GAME OVER. It was " + secret.getSecret());
} else {
out.printLine(str + " ---> " + correctPosition + " at correct pos. " + incorrectPosition + " at incorrect pos." + attempts + " attemps left.");
}
}
}
// 3. Game close
in.readString();
System.exit(0);
}
}
But, now, the input needs a string instead of just a character. That's why we need to extend the IInput interface.
There are several options…
- Modify the interface and implement the methods in all the classes that implement it.
- Create a new interface that extends the previous one (new version).
- Create a new interface not related with the previous one.
- Others that more experienced people than me can suggest in the comments ?
Option 1 breaks the existing code but at the moment it is very easy to implement a new method and I don't want to create versions of just a simple interface.
package manager;
public interface IInput {
char readChar();
String readString();
}
Finally a couple of screenshots of the mini-game
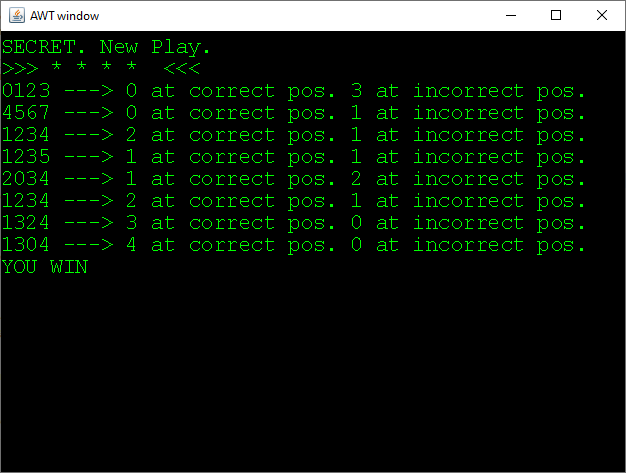
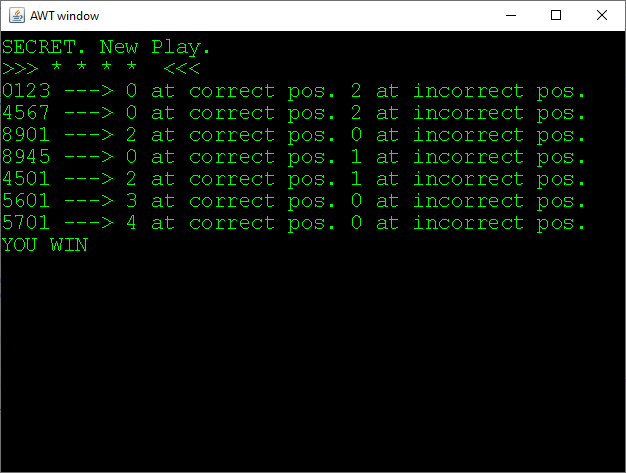