Greetings everyone!
I am trying to create a simple DOOM clone. I got some 8 directional sprites, which I want to draw in 3D dimentional space. I have an enemy class which has a facing direction. I also have a player with a camera. I want for the sprite to choose the correct direction depending on its facing direction and relative to the camera's viewing direction. I.e if I start going around in circles around the sprite it should rotate as well.
I want to achieve the same thing as in this animated picture:
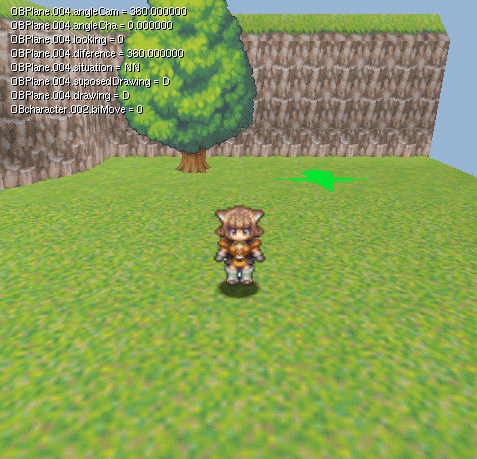
This is what I have tried so far, but it doesn't work correctly all of the time. Only for certain angles.
// Inside the enemy class
vec3 playerToSprite = this - > position - player.position;
vec3 direction = this - > facing_direction - playerToSprite;
angle = radtodeg(arctan2(direction.x, direction.y));
if (angle > 90) {
angle = 450 - angle;
} else {
angle = 90 - angle;
}
// Choose the correct sprite. Sprites 0 is the East facing sprite, 1 is the NorthEast, 2 is North and it goes on clockwise
this - > sprite = sprites[angle / 45];
Can you help me to fix my code?
EDIT: I managed to solve my problem. Here is the complete solution:
// Camera facing direction (in 2D)
// Direction is the direction at which the camera is looking at ( in 2D )
vec2 camera = vec2(cos(degtorad(CCamera::GetMainCamera() - > transform.direction)), -sin(degtorad(CCamera::GetMainCamera() - > transform.direction)));
// Object facing direction (in 2D)
// direction is the facing direction of the sprite, i.e. the direction at which the sprite is Looking At. ( in 2D )
vec2 facing = vec2(cos(degtorad(self - > transform - > direction)), -sin(degtorad(self - > transform - > direction)));
// Dot product
double cosine = dot(camera, facing);
double angle = radtodeg(arctan2(facing.y, facing.x) - arctan2(camera.y, camera.x));
if (angle > 90.0) {
angle = 450.0 - angle;
} else {
angle = 90.0 - angle;
}
self - > sprite = sprite_set[floor(angle / 45.0)];