Hi, I have enabled pcf filtering in my shader yet it doesnt work well. I dont get soft shadows. How can I address this issue?
float2 offset(int u, int v, float sm_size)
{
return float2(u * (1.0f / sm_size), v * (1.0f / sm_size));
}
float SampleDepthMap(Texture2D texArr[3], int index, float2 coord, float lightDepthValue)
{
float ret = 0;
if(index == 0)
{
for (float y = -1.5; y <= 1.5; y += 1.0)
{
for (float x = -1.5; x <= 1.5; x += 1.0)
{
ret += texArr[0].SampleCmpLevelZero(ssModelCSM, coord + offset(x, y, 4096), lightDepthValue);
}
}
}
else if(index == 1)
{
for (float y = -1.5; y <= 1.5; y += 1.0)
{
for (float x = -1.5; x <= 1.5; x += 1.0)
{
ret += texArr[1].SampleCmpLevelZero(ssModelCSM, coord + offset(x, y, 2048), lightDepthValue);
}
}
}
else if (index == 2)
{
for (float y = -1.5; y <= 1.5; y += 1.0)
{
for (float x = -1.5; x <= 1.5; x += 1.0)
{
ret += texArr[2].SampleCmpLevelZero(ssModelCSM, coord + offset(x, y, 2048), lightDepthValue);
}
}
}
ret /= 16.0f;
return ret;
}
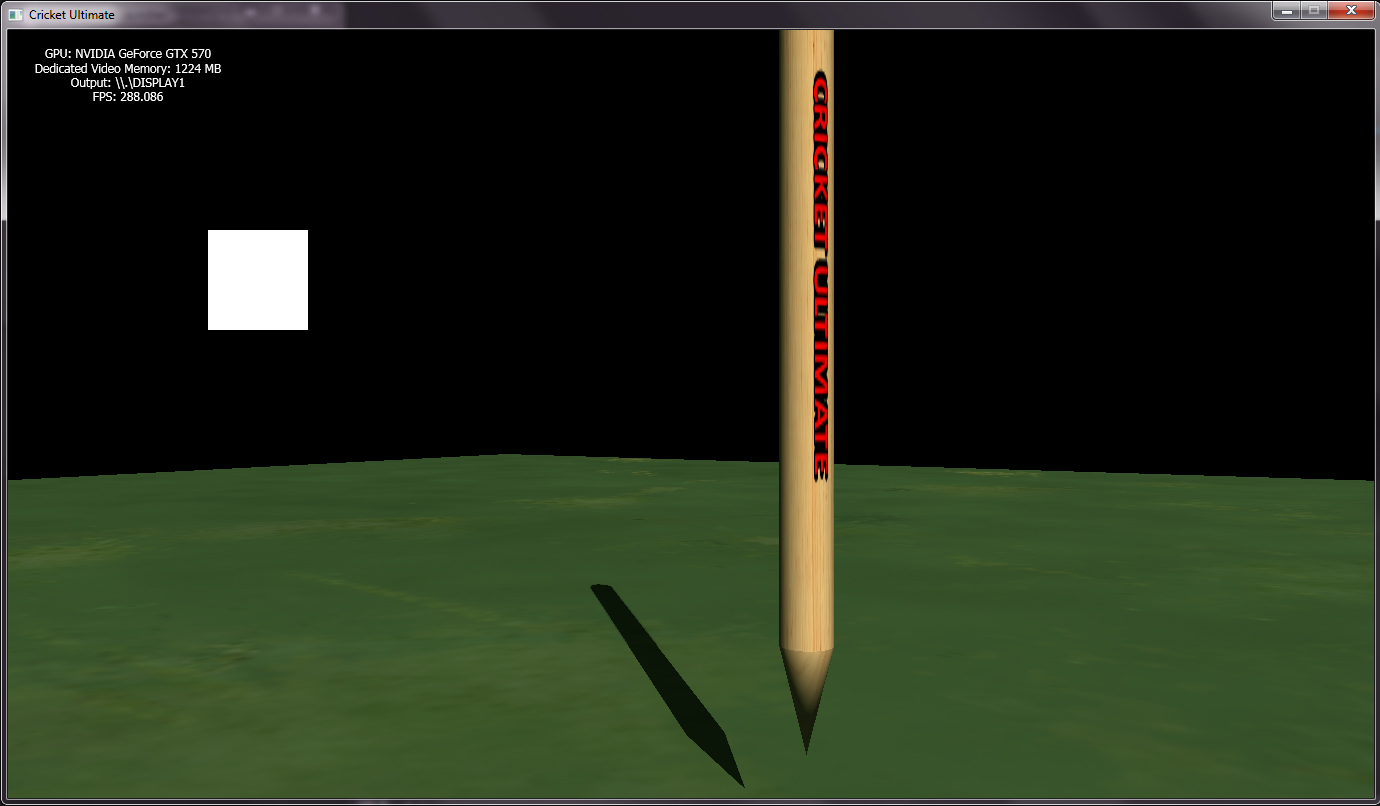