I’m currently working on a project involving animation programming using OpenGL and BVH (Biovision Hierarchy) data for animation, and I am trying to get my head around it. I’ve run into some issues and could use some insight.
The problem I’m facing is that when I render my animation, it doesn’t come out as expected. I find the format of BVH quite confusing but this seems correct and it should work but the animation looks quite odd.
I am unsure if, for each rotation you need to multiply it by the parentMatrix? doing this seemed to prevent the legs going up to the up and flicking back down so I assumed it was correct?
BVH file: https://pastebin.com/4w2Qv5TD
void Skeleton::drawJoint(Mat4& viewMatrix, Mat4 parentMatrix, Joint* joint, uint32_t currFrame)
{
vec3 offset_from_parent = vec3(
joint->jointOffsetX,
joint->jointOffsetY,
joint->jointOffsetZ
);
vec3 anotherRot = vec3(
frames[currFrame][BVH_CHANNEL[joint->joint_channel[0]]],
frames[currFrame][BVH_CHANNEL[joint->joint_channel[1]]],
frames[currFrame][BVH_CHANNEL[joint->joint_channel[2]]]
);
auto jointPos = parentMatrix * (offset_from_parent);
// get rotation for current joint for current frame
auto boneRot = parentMatrix * bone_rotations[currFrame][joint->id];
// BVH stores rotation as Z,Y,X so, boneRot.x would be rotation for z
auto z = boneRot.x;
auto y = boneRot.y;
auto x = boneRot.z;
//z,y,x multiply like: x,y,z
auto local = Mat4::Translate(jointPos) * (Mat4::RotateX(x) * Mat4::RotateY(y) * Mat4::RotateZ(z));
for(auto& child : joint->Children)
{
vec3 end = local * vec3(child.joint_offset[0], child.joint_offset[1], child.joint_offset[2]);
drawCylinder(viewMatrix, jointPos, end);
drawJoint(viewMatrix, localMatrix, &child, scale, frame);
}
}
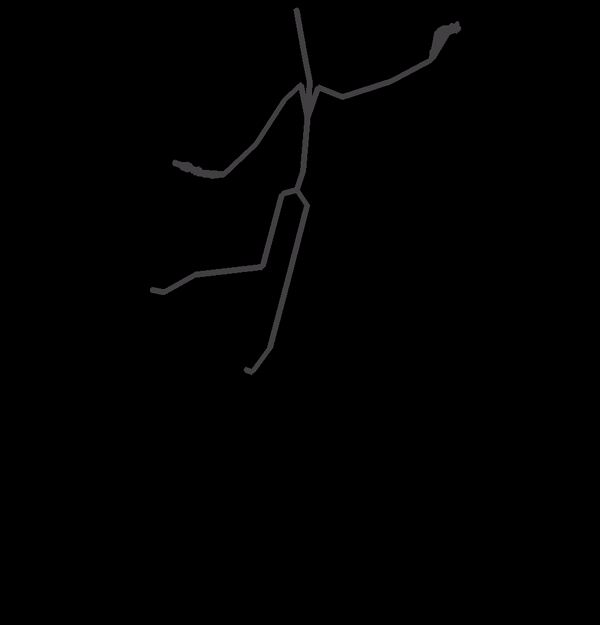