Hi There,
I'm trying to draw a spritesheet on screen which contains 16x16 sprites evenly spaced with a 1px gap between each, however I'm struggling to get the math right in order to take into account the spacing between pixels when working out which sprite needs to be drawn.
The current result I have looks like this:
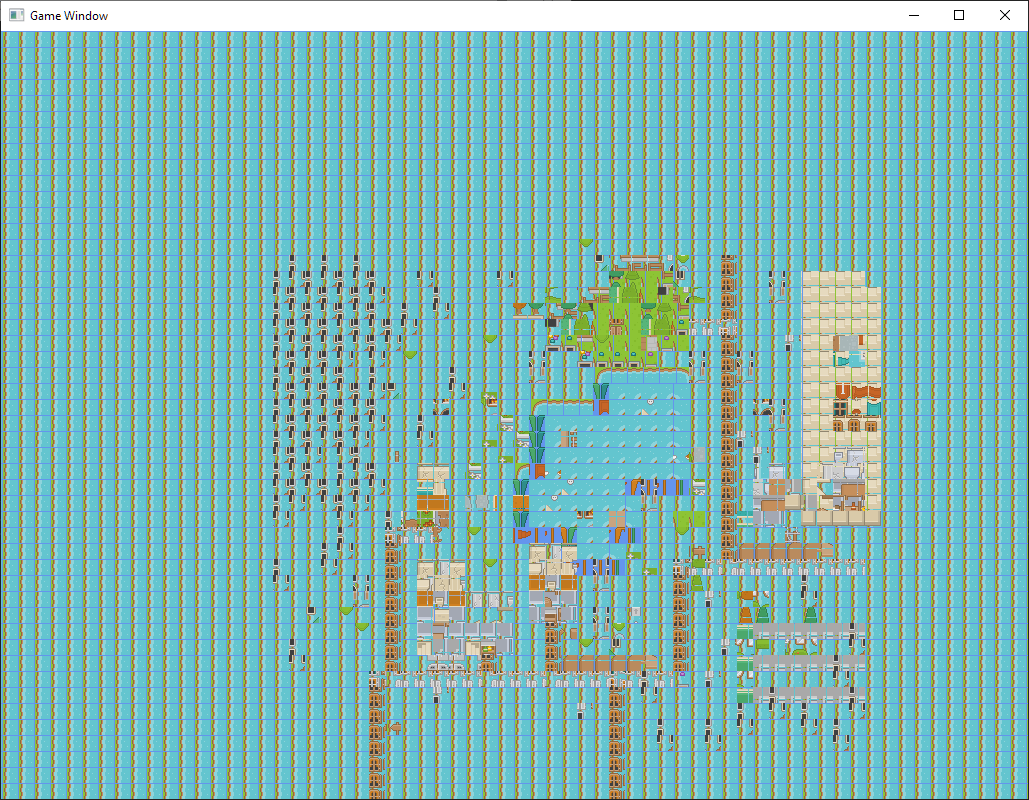
However it should look something like this:
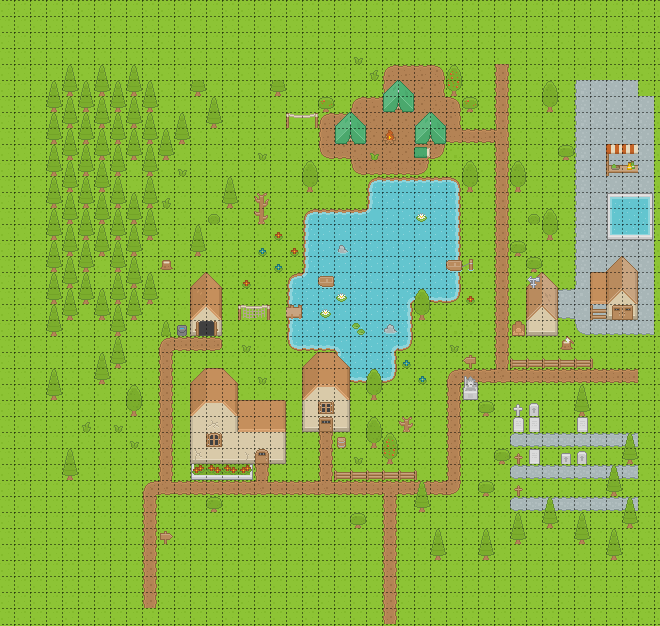
The issue is because I only have a ID number that corresponds to the sprite that needs to be drawn on screen (The ID corresponds to a specific sprite on the sheet, starting at 0 and incrementing up to the total number of sprites on the sheet). The library I'm using to load the level works out the sprite position on the sprite sheet using this ID number as follows:
// I've hard-coded the values below for easy reading. Usually these are worked out at runtime.
int spritesheet_width = 968;
int spritesheet_height = 526;
int sprite_width = 16;
int sprite_height = 16;
int sprite_id = 110; // During the draw-loop this updates with the ID of the next sprite to draw.
int sprite_X = (sprite_id % (spritesheet_width / sprite_width) * sprite_width;
int sprite_Y = (sprite_id / (spritesheet_width / sprite_width) * sprite_height;
DrawSprite(sprite_X, sprite_Y ...);
I believe this isn't taking into account the spacing between sprites which results in the broken on-screen view. Is there a way I can adjust this math to take the spacing between sprites into account? So far the various options I've tried haven't worked (My math skills are dreadful!). Short of removing all of the spacing from the spritesheet and re-exporting the level, I'm out of ideas.
Thanks in advance!