Hi, I'm facing this issue of properly settings up my textures for the object. Since I'm new to all these. Can you guys tell me how to do that? D3D11 is pretty easy. D3D12, here's my code.
D3D12_DESCRIPTOR_HEAP_DESC srvHeapDesc = {};
srvHeapDesc.NumDescriptors = mTexCount;
srvHeapDesc.Type = D3D12_DESCRIPTOR_HEAP_TYPE_CBV_SRV_UAV;
srvHeapDesc.Flags = D3D12_DESCRIPTOR_HEAP_FLAG_SHADER_VISIBLE;
ThrowIfFailed(md3dDevice->CreateDescriptorHeap(&srvHeapDesc, IID_PPV_ARGS(&mSrvHeap)));
=================================================================
CD3DX12_ROOT_PARAMETER slotRootParameter[2];
CD3DX12_DESCRIPTOR_RANGE cbvTable;
cbvTable.Init(D3D12_DESCRIPTOR_RANGE_TYPE_CBV, 1, 0);
CD3DX12_DESCRIPTOR_RANGE srvTable;
srvTable.Init(D3D12_DESCRIPTOR_RANGE_TYPE_SRV, 1, 0);
slotRootParameter[0].InitAsDescriptorTable(1, &cbvTable);
slotRootParameter[1].InitAsDescriptorTable(1, &srvTable, D3D12_SHADER_VISIBILITY_PIXEL);
D3D12_STATIC_SAMPLER_DESC sampler = {};
sampler.Filter = D3D12_FILTER_ANISOTROPIC;
sampler.AddressU = D3D12_TEXTURE_ADDRESS_MODE_WRAP;
sampler.AddressV = D3D12_TEXTURE_ADDRESS_MODE_WRAP;
sampler.AddressW = D3D12_TEXTURE_ADDRESS_MODE_WRAP;
sampler.MipLODBias = 0;
sampler.MaxAnisotropy = 16;
sampler.ComparisonFunc = D3D12_COMPARISON_FUNC_NEVER;
sampler.BorderColor = D3D12_STATIC_BORDER_COLOR_TRANSPARENT_BLACK;
sampler.MinLOD = 0.0f;
sampler.MaxLOD = D3D12_FLOAT32_MAX;
sampler.ShaderRegister = 0;
sampler.RegisterSpace = 0;
sampler.ShaderVisibility = D3D12_SHADER_VISIBILITY_PIXEL;
// A root signature is an array of root parameters.
CD3DX12_ROOT_SIGNATURE_DESC rootSigDesc(2, slotRootParameter, 1, &sampler,
D3D12_ROOT_SIGNATURE_FLAG_ALLOW_INPUT_ASSEMBLER_INPUT_LAYOUT);
// create a root signature with a single slot which points to a descriptor range consisting of a single constant buffer
ComPtr<ID3DBlob> serializedRootSig = nullptr;
ComPtr<ID3DBlob> errorBlob = nullptr;
HRESULT hr = D3D12SerializeRootSignature(&rootSigDesc, D3D_ROOT_SIGNATURE_VERSION_1,
serializedRootSig.GetAddressOf(), errorBlob.GetAddressOf());
===========================================================================
void RESrcLoader::BuildTextureSRVs()
{
int i = 0;
for (auto &it : mTextures)
{
auto tex = it.second;
CD3DX12_CPU_DESCRIPTOR_HANDLE srvCPUHeapHandle(mSrvHeap->GetCPUDescriptorHandleForHeapStart());
CreateShaderResourceView(md3dDevice.Get(), tex.Get(), srvCPUHeapHandle);
srvCPUHeapHandle.Offset(mCbvSrvUavDescriptorSize);
mList[it.first] = i++;
}
}
==============================================================================
draw code
mCommandList->SetGraphicsRootSignature(mRootSignature.Get());
{
ID3D12DescriptorHeap* ppHeaps[] = { mCbvHeap.Get()};
mCommandList->SetDescriptorHeaps(_countof(ppHeaps), ppHeaps);
mCommandList->SetGraphicsRootDescriptorTable(0, mCbvHeap->GetGPUDescriptorHandleForHeapStart());
}
{
ID3D12DescriptorHeap* ppHeaps[] = { mSrvHeap.Get() };
mCommandList->SetDescriptorHeaps(_countof(ppHeaps), ppHeaps);
}
for (auto md : Model)
{
CD3DX12_GPU_DESCRIPTOR_HANDLE srvGPUHandle(mSrvHeap->GetGPUDescriptorHandleForHeapStart());
srvGPUHandle.Offset(mList[md.mMeshTextureName], mCbvSrvUavDescriptorSize);
mCommandList->SetGraphicsRootDescriptorTable(1, srvGPUHandle);
md.DrawMesh(mCommandList);
}
this model contains single texture. it works but multiple ones are not
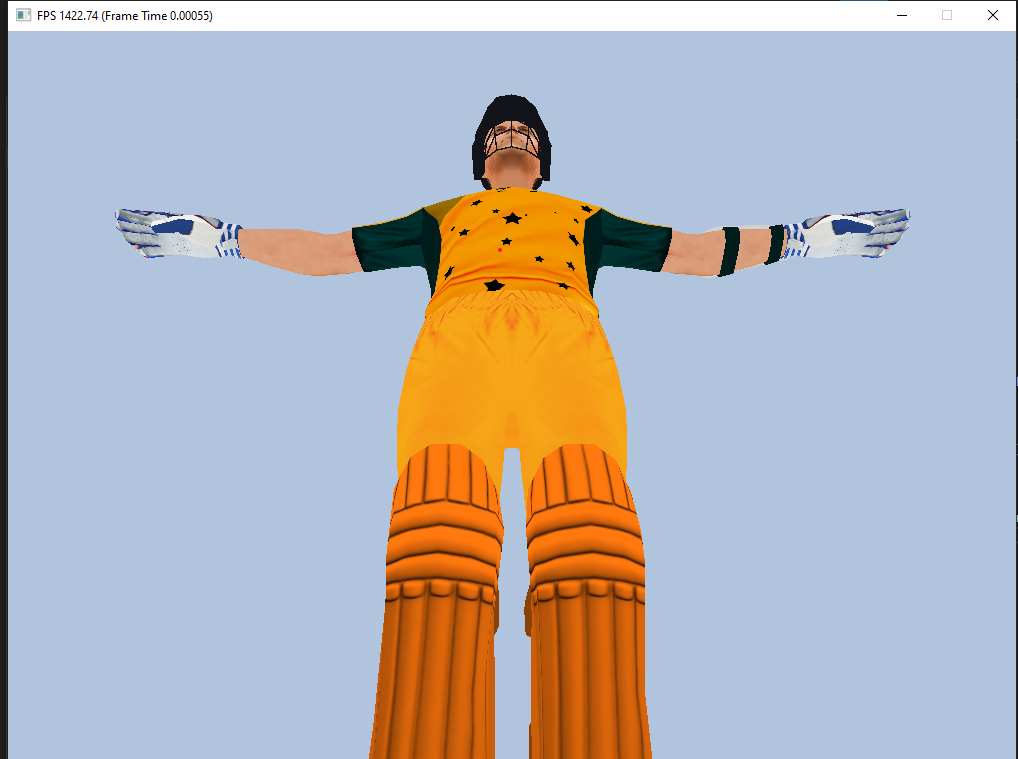